📲 Telegram Gateway API Client
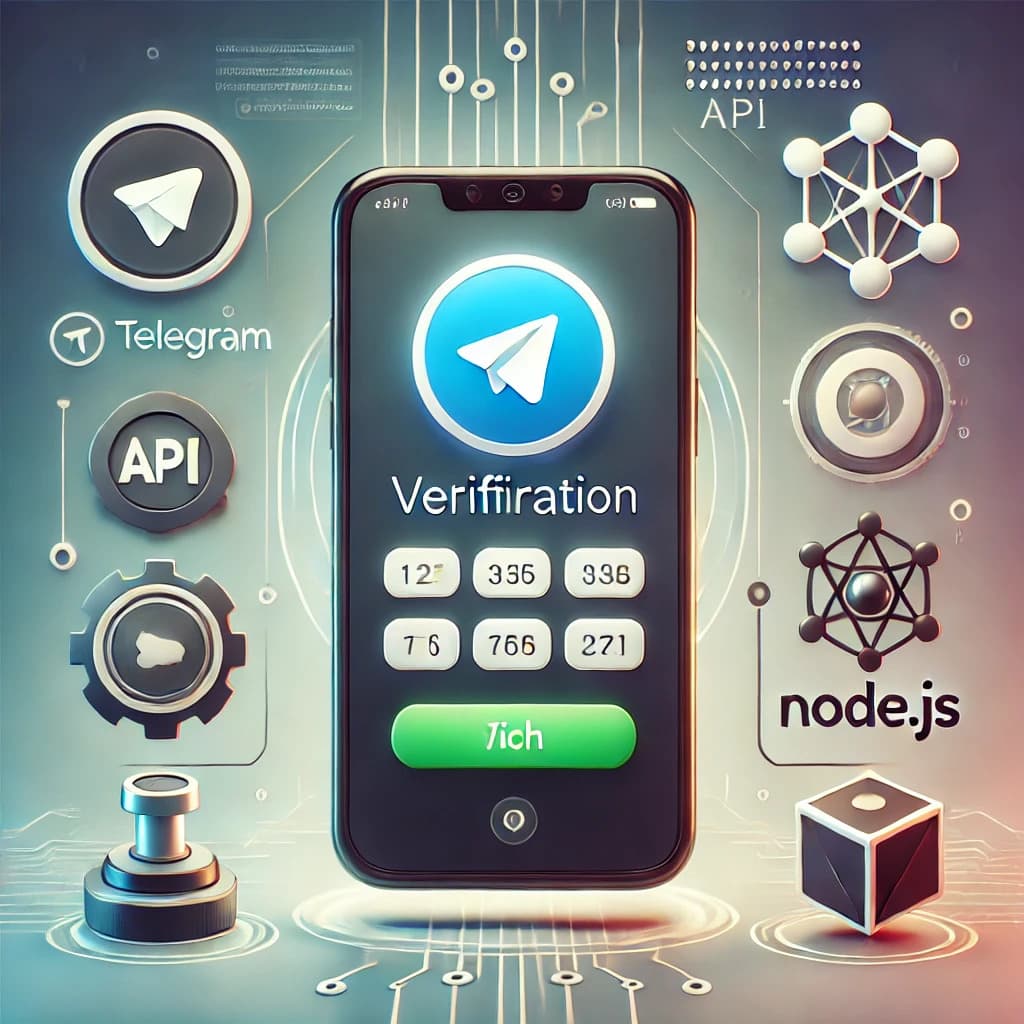
As developers, we often deal with tasks like verifying phone numbers. While powerful APIs like the Telegram Gateway API exist, the complexity of managing requests can slow us down.
That’s why I created node-telegram-gateway-api
, a Node.js client to make phone verification simple, efficient, and developer-friendly.
In this post, I’ll walk you through the library’s features, share how it works, and discuss the thought process behind building it.
🛠️ Why I Built This Library
Verification workflows can be tedious. Sending codes, checking their status, and managing revocations require repetitive code and careful handling of API interactions. I wanted to create a library that:
- Abstracts the complexity of Telegram Gateway API calls.
- Provides clear and robust documentation.
- Offers developers a clean, intuitive API to work with.
With this in mind, I created node-telegram-gateway-api
.
📦 Installation and Setup
Getting started with the library is straightforward. Install the package using npm:
npm install node-telegram-gateway-api
Next, you’ll need an API key from the Telegram Gateway API. Here’s a quick example of how to initialize the client:
import { TelegramGateway } from "node-telegram-gateway-api";
const apiKey = "YOUR_TELEGRAM_API_KEY";
const client = new TelegramGateway(apiKey);
âś… Type Safety with TypeScript
node-telegram-gateway-api
ensures type safety using TypeScript. The library provides typed API responses and validates parameters at compile time, reducing runtime errors. TypeScript support helps you catch issues early and boosts development efficiency with features like autocomplete.
For instance, when sending a verification message, TypeScript ensures that all parameters are correctly typed:
const response = await client.sendVerificationMessage("+1234567890", {
sender_username: "YourApp", // string
code_length: 6 // number
});
🚀 Key Features and Examples
1. Sending Verification Messages
Sending a verification code is as simple as calling a single method:
async function sendVerification() {
const response = await client.sendVerificationMessage("+1234567890", {
sender_username: "YourApp",
code_length: 6,
});
if (response.ok) {
console.log("Message sent successfully:", response.result);
} else {
console.error("Error sending message:", response.error);
}
}
This method lets you configure options like the sender’s username and the length of the code, making it flexible for different use cases.
2. Checking Send Ability
Before you send a message, verify if it’s possible to send a code to a specific number:
async function checkSendAbility() {
const response = await client.checkSendAbility("+1234567890");
if (response.ok) {
console.log("Able to send verification message:", response.result);
} else {
console.error("Cannot send message:", response.error);
}
}
This feature is great for pre-validating phone numbers.
3. Tracking Verification Status
After sending a verification message, you can track its status using:
async function checkVerificationStatus() {
const response = await client.checkVerificationStatus(
"request_id_here",
"user_entered_code"
);
if (response.ok) {
console.log("Verification status:", response.result);
} else {
console.error("Error checking status:", response.error);
}
}
This ensures you always know whether a code has been successfully verified.
4. Revoking Verification Messages
Finally, you can revoke a verification message if necessary:
async function revokeMessage() {
const response = await client.revokeVerificationMessage("request_id_here");
if (response.ok) {
console.log("Message revoked successfully");
} else {
console.error("Error revoking message:", response.error);
}
}
🌟 Lessons Learned and Challenges
Building node-telegram-gateway-api
was both challenging and rewarding.
Some key takeaways include:
- API Design: Striking the right balance between flexibility and simplicity is crucial.
- Error Handling: Comprehensive error handling is essential for working with external APIs.
- Documentation: Clear, concise documentation can make or break a project’s usability.
🛠️ Get Involved!
I believe in the power of open source. If you find node-telegram-gateway-api
useful or have ideas to improve it, contributions are always welcome! Head over to the GitHub repo to get started.
🎉 Conclusion
node-telegram-gateway-api
simplifies phone verification processes, saving developers time and effort. I hope this library proves helpful for your projects, and I’m excited to see how it evolves with the community’s feedback.
Want to dive deeper? Check out the npm page or explore the GitHub repository.
Feel free to share your thoughts or issues with me! 🚀